The Griffins
By Stephen Swetonic, Weston Goggins, and Connor Goan
March 15th, 2021
Window API Project
This webiste documents the API for a basic window object, a Draggable object, and a Resizable object that add their named functionality. Class definitions, example code, and demos are presented here to demonstrate this project. WindowManager and Window allow the creation of in-game windows, which are a combination of renderable borders and a camera. The Draggable class allows windows and other objects to be dragged with the mouse. The Resizeable class allows windows and other objects to be dynamically resized with the mouse.
The window object is associated with it’s own camera, allowing the developer to present what they wish within the window while allowing that window to keep the same position relative to the canvas, not the main camera. This window will also have the options to be dragged around the screen and resized with the mouse if the developer wishes to enable them
Demonstration of our project: DEMO
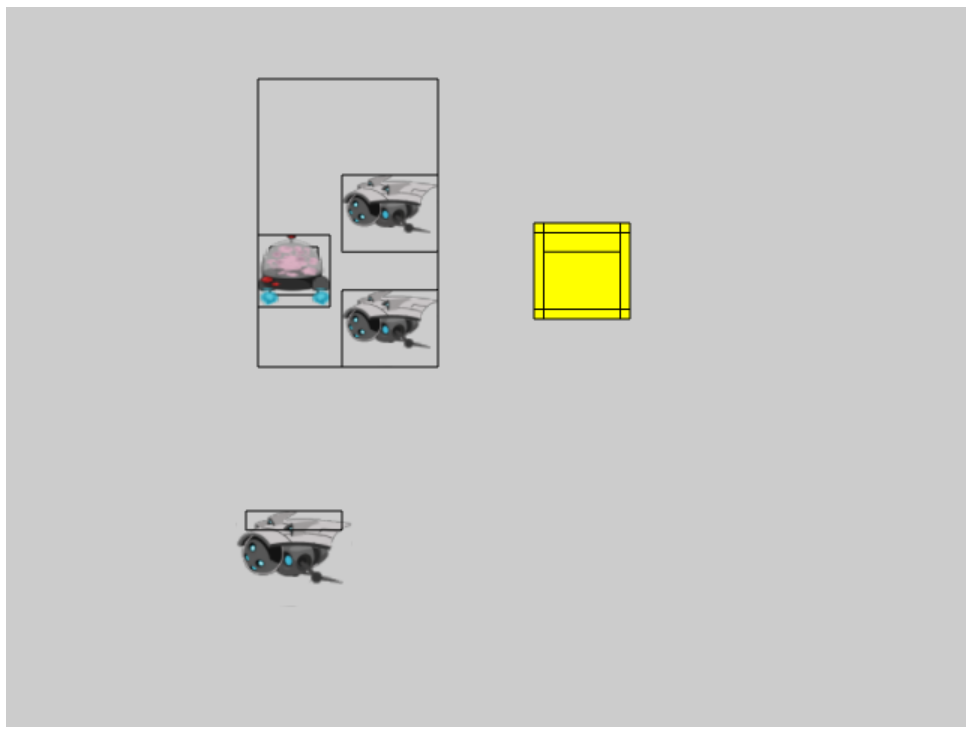
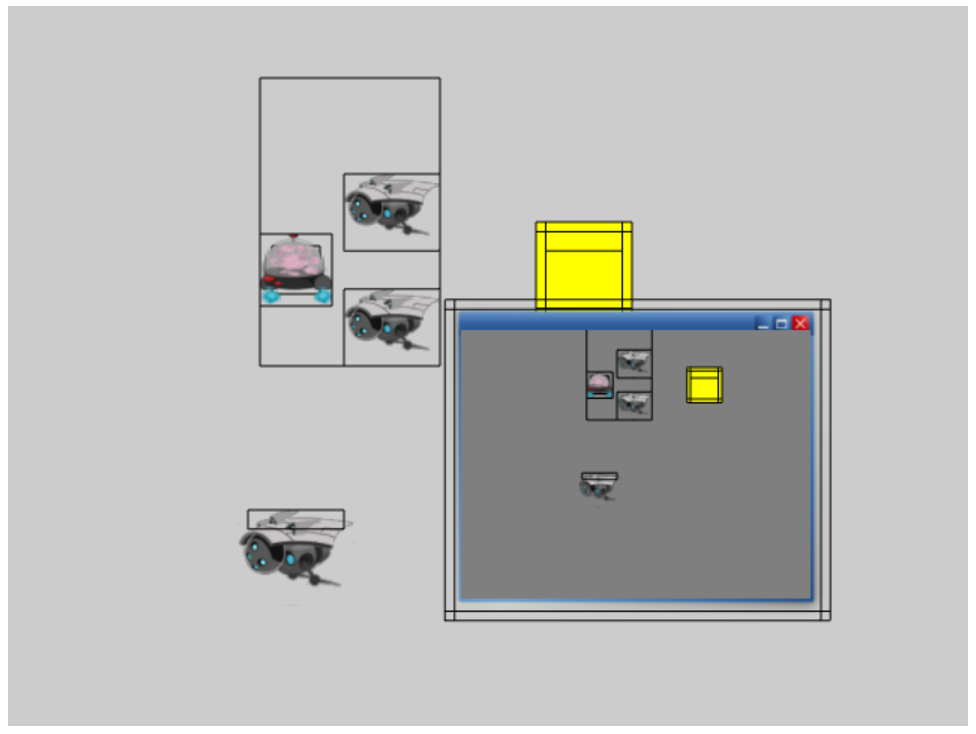
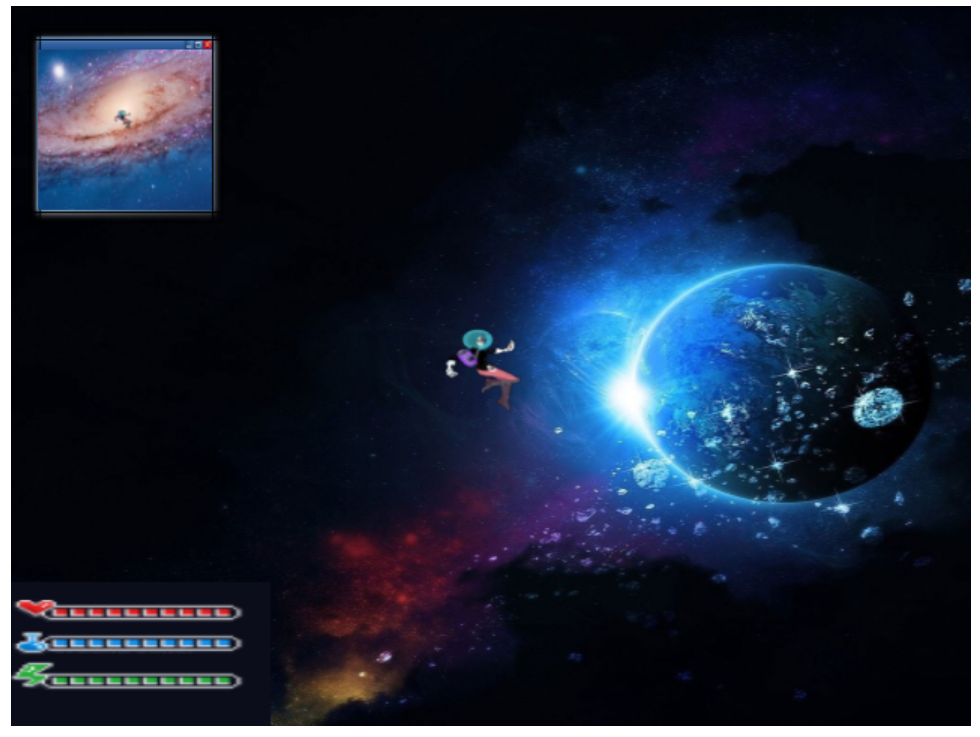
Window
Constructor
Window(renderableObject, windowCam, worldCam, xoffsetleft, xoffsetright, yoffsetbottom, yoffsettop, drag, resize)
PARAMETERS
Name | Type | Description |
---|---|---|
renderableObject | Renderable | Any Renderable or subclass of Renderable (i.e. SpriteAnimateRenderable) |
windowCam | Camera Object | The camera for the window. Dimensions will not stay the same. |
worldCam | Camera Object | The world camera that the window will be drawn upon |
xoffsetleft | number | Offset in pixels from the left side of the canvas |
xoffsetright | number | Offset in pixels from the right side of the canvas |
yoffsetbottom | number | Offset in pixels from the bottom of the canvas |
yoffsettop | number | Offset in pixels from the top of the canvas |
drag | Booolean | True to enable dragging functionality of the window. False to disable. |
resize | Boolean | True to enable dragging resizing of the window. False to disable. |
Initialize
initialize()
Initializes the draggable and resizable versions of renderable if enabled.
setdragarea
setDragArea(width, height)
Defines the dimensions of the drag area. The drag area is position to be at the top of the window.
Name | Type | Description |
---|---|---|
width | Number | Width of the drag area in WC |
height | Number | Height of the drag area in WC |
setmouseposition
setMousePosition(x, y)
Used to pass the mouse position to this object.
Name | Type | Description |
---|---|---|
x | Number | Camera's WC X position |
y | Number | Camera's WC Y position |
setISDrag
setISDrag(bool)
Defines if the window is draggable or not, depending on whether the input is true or false.
Name | Type | Description |
---|---|---|
bool | bool | A boolean that allows the window to be draggable if set to true. |
setISResize
setISResize(bool)
Defines if the window is resizeable or not, depending on whether the input is true or false
Name | Type | Description |
---|---|---|
bool | bool | A boolean that allows the window to be resizable if set to true. |
getCamera
getCamera()
Returns the window's camera.
setkey
setKey()
Sets the window's key.
Name | Type | Description |
---|---|---|
key | number | The window's numerical key (ID). |
getkey
getKey()
Returns the window's key.
draw
draw(cam, objects)
Draws the window on the inputted camera, and then draws the objects within the window on the inputted list of objects.
Name | Type | Description |
---|---|---|
cam | Camera | The world camera that this window will be displayed on. |
objects | Array | An array of objects to be displayed within the window's camera./td> |
update
update(cam)
Draws the window object. Its dimensions are specified by the inputted world camera.
Name | Type | Description |
---|---|---|
cam | Camera | The world camera that the window's dimensions will compare to. |
Window Creation Tutorial
Creating a single window object with a texture is relatively simple. In this example, we assume this.mCamera was already defined. We create a new Texture Renderable with the image below. Then create a camera to associate with the Window. The Window object is then initialized with that TextureRenderable and Camera with the dragging flag enabled.
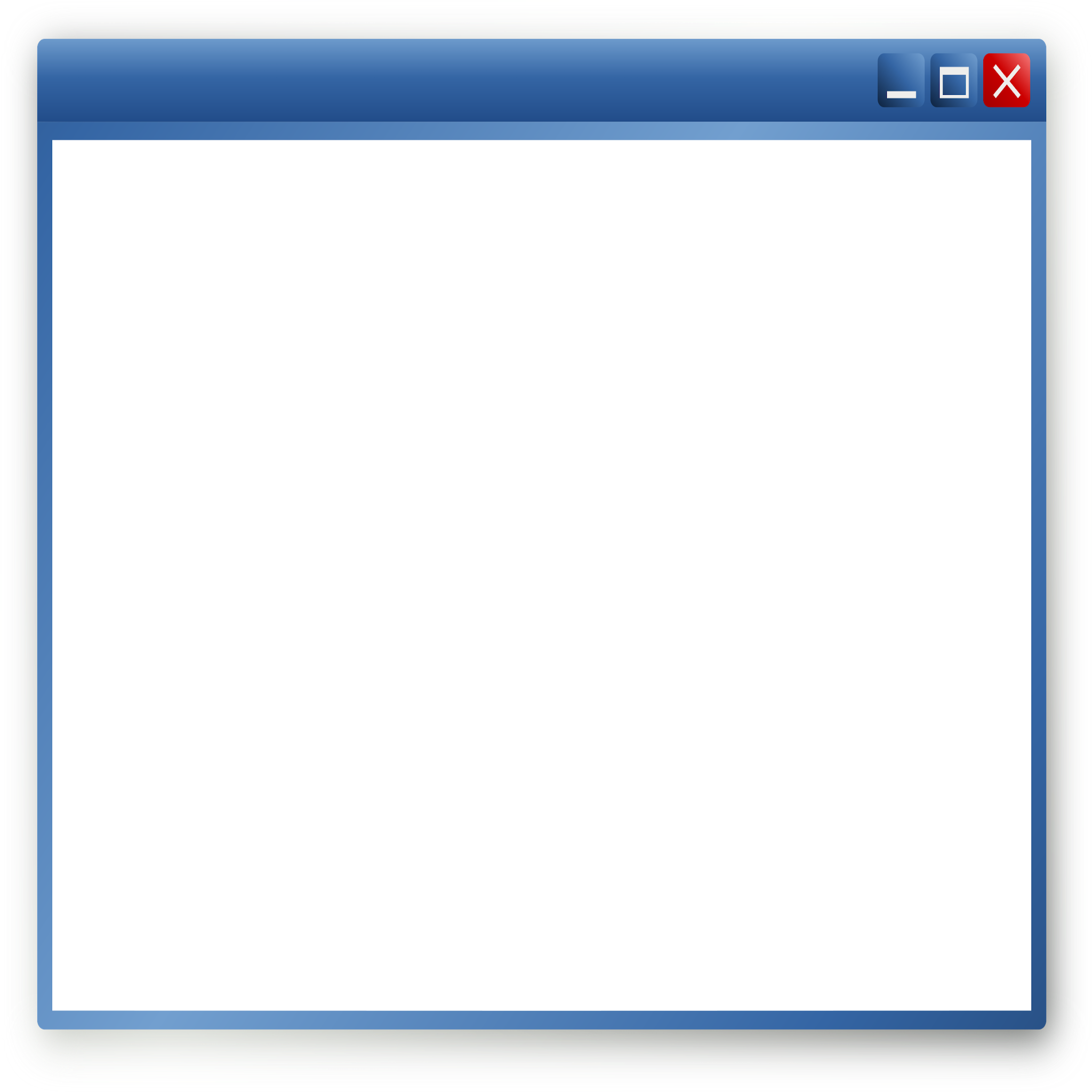
MyGame.prototype.initialize = function() {
this.mWindows = new WindowManager();
// Create TextureRenderable
this.box = new TextureRenderable(this.kWindowSprite);
this.box.setColor([1, 1, 1, 0]);
this.box.getXform().setPosition(this.mCamera.getWCCenter()[0] + ((Math.random() * 80) - 40),
this.mCamera.getWCCenter()[1] + ((Math.random() * 60) - 30));
this.box.getXform().setSize(20, 15);
// Create a camera for the window
var cam = new Camera(vec2.fromValues(30, 27.5), // position of the camera
20, // width of camera
[0, 0, 0, 0] // viewport (orgX, orgY, width, height)
);
cam.setBackgroundColor([0.5, 0.5, 0.5, 1]);
// Create a Window, set drag area, and add to window manager
var window = new Window(this.box, cam, this.mCamera, 1, //Left offset
1, //Right offset
1.2, //Bottom offset
1.6, //Top offset
true, false);
window.initialize();
window.setDragArea(17.5, 5);
this.mWindows.add(window, true);
}
In this example of the MyGame update() and draw() function, the collection of objects (this.mDrawnObjects) contains all the objects we want to draw. These are drawn to the main camera and passed into the draw function of our WindowManager, which also uses the main camera. Note that setMousePosition() does not need to be called here, it is called from WindowManager.update().
MyGame.prototype.update = function() {
this.mWindows.update(this.mCamera);
}
MyGame.prototype.draw = function () {
// Step A: clear the canvas
gEngine.Core.clearCanvas([0.9, 0.9, 0.9, 1.0]); // clear to light gray
this.mCamera.setupViewProjection();
for (var i = 0; i < this.mDrawnObjects.length; i++) {
this.mDrawnObjects[i].draw(this.mCamera);
}
this.mWindows.draw(this.mCamera, this.mDrawnObjects);
};
Window Manager
Constructor
WindowManager()
Defines a WindowManager object to store a set of windows, update, and call them all at once. Additionally, the WindowManager can push a window to the front to be drawn first.
Initialize
initialize()
Initializes the window key to 0.
add
add(window, front)
Adds a window to the front of the list if 'front' is true, otherwise its added to the back.
Name | Type | Description |
---|---|---|
window | Window | The window to be added to the window list. |
front | bool | If set to true, will layer the new window on top of all existing windows. Otherwise will be pushed to the back layer |
remove
remove(window)
Removes the inputted window from the list.
Name | Type | Description |
---|---|---|
window | Window | The window to be removed the window list. |
pushtofront
pushToFront(window)
Pushes the inputted window to the fron to list, layering it on top of other windows in the list.
Name | Type | Description |
---|---|---|
window | Window | The window to be pushed in front of all other windows. |
draw
draw(cam, objects)
Draws all windows in the inputted camera, and all windows display the inputted list of objects.
Name | Type | Description |
---|---|---|
cam | Camera | The world camera that all windows will be drawn upon. |
objects | Array of Renderables | An array of objects that the window cameras will draw. |
update
update(cam)
Draws all windows in the inputted camera, and all windows display the inputted list of objects.
Name | Type | Description |
---|---|---|
cam | Camera | The world camera that all windows will be drawn upon. |
Draggable
A class that allows a renderable to be moved by clicking and dragging the left mosue button
Constructor
Draggable(renderableObject)
Defines a Draggable and is passed a Renderable object.
Name | Type | Description |
---|---|---|
renderableObject | Renderable | Any Renderable or subclass of Renderable (i.e. SpriteAnimateRenderable) |
draw
draw(camera)
Draws the renderable and the borders to the camera, if enabled.
Name | Type | Description |
---|---|---|
camera | Camera | Reference to the main world camera |
update
update()
Detects clicks in the drag area and changes renderable transform accordingly. The renderable will move with the mouse position after a left-menu click in the drag area and will stop when the mouse button is released.
setmouseposition
setMousePosition(x, y)
Used to pass the mouse position to this object.
Name | Type | Description |
---|---|---|
x | Number | Camera's WC X position |
y | Number | Camera's WC Y position |
setdragarea
setDragArea(xOffset, yOffset, width, height)
Define the position and size of the drag area by WC offset from renderable's center.
Name | Type | Description |
---|---|---|
xOffset | Number | X Offset in WC from renderable center position |
yOffset | Number | Y Offset in WC from renderable center position |
width | Number | Width of the drag area in WC |
height | Number | Height of the drag area in WC |
enabledragareaborder
enableDragAreaBorder()
Shows drag area borders
disabledragareaborder
disableDragAreaBorder()
Disable drag area borders
getxform
getXform()
Returns a reference to the Renderable's transform.
Creating a Draggable Object
A draggable only needs a single Renderable passed to it. This can be a basic Renderable, TextureRenderable, SpriteAnimateRenderable, or anything with an update and draw function.
MyGame.prototype.initialize = function () {
// Step A: set up the cameras
this.mCamera = new Camera(
vec2.fromValues(30, 30), // position of the camera
100, // width of camera
[0, 0, 640, 480] // viewport (orgX, orgY, width, height)
);
this.mCamera.setBackgroundColor([0.8, 0.8, 0.8, 1]);
// sets the background to gray
// Create a SpriteAnimateRenderable
this.mSpriteAnimate = new SpriteAnimateRenderable(this.kSpriteSheet);
this.mSpriteAnimate.setColor([1, 1, 1, 0]);
this.mSpriteAnimate.getXform().setPosition(this.mSpawnX, this.mSpawnY);
this.mSpriteAnimate.setAnimationType(SpriteAnimateRenderable.eAnimationType.eAnimateRight);
this.mSpriteAnimate.setAnimationSpeed(50);
this.mSpriteAnimate.getXform().setPosition(10, 10);
this.mSpriteAnimate.getXform().setSize(12, 10);
this.mSpriteAnimate.setSpriteSequence(512, 0, // first element pixel position: top-left 512 is top of image, 0 is left of image
204, 164, // widthxheight in pixels
5, // number of elements in this sequence
0);
// Create a Draggable and pass in our SpriteAnimateRenderable
this.mDragTest = new Draggable(this.mSpriteAnimate, this.mCamera);
this.mDragTest.setDragArea(0, 4, 10, 2);
};
In our update function, we only need to call Draggable's update function and call setMousePosition(x, y) so the object gets the current mouse position. Don't forget to call the SpriteAnimateRenderable's update animation function so it animates. Drawing is similarly simple, just call draw on the Draggable.
MyGame.prototype.update = function() {
this.mDragTest.setMousePosition(this.mCamera.mouseWCX(), this.mCamera.mouseWCY());
this.mSpriteAnimate.updateAnimation();
this.mDragTest.update();
};
MyGame.prototype.draw = function () {
this.mDragTest.draw(this.mCamera);
}
Resizable
A class that allows a renderable to be resized by dragging the edges with the mouse
Constructor
Resizeable(renderableObject)
Defines a Resizeable, the renderableObject is the object Resizeable will be applied to. Borders of resize area are on by default.
Name | Type | Description |
---|---|---|
renderableObject | Renderable | Any Renderable or subclass of Renderable (i.e. SpriteAnimateRenderable) |
initialize
initialize()
Initializes the Resizable.
draw
draw(camera)
Draws the renderable and the borders to the camera, if enabled.
Name | Type | Description |
---|---|---|
camera | Camera | Reference to the main world camera |
update
update()
Detects clicks and changes renderable transform accordingly.
setmouseposition
setMousePosition(x, y)
Used to pass the mouse position to this object.
Name | Type | Description |
---|---|---|
x | Number | Camera's WC X position |
y | Number | Camera's WC Y position |
enableresizeareaborder
enableResizeAreaBorder()
Shows resize borders
disableresizeareaborder
disableResizeAreaBorder()
Disable resize borders
Creating a Resizable Object
Resizable is almost exactly the same as Draggable, just different functionality. The object takes a Renderable (or subclass) and makes it resizable on every edge and corner. The resize bounds are enabled by default, but can be controlled with disable()/enable().
MyGame.prototype.initialize = function () {
// Create a renderable
this.mRenderableTest = new Renderable();
this.mRenderableTest.setColor([1, 0, 0, 1]);
this.mRenderableTest.getXform().setPosition(60, 27.5);
this.mRenderableTest.getXform().setSize(30, 30);
// Create a resizable and pass in renderable
this.mResizeTest = new Resizeable(this.mRenderableTest, this.mCamera);
this.mResizeTest.initialize();
}
The Resizable's update and draw functions need only be called from the client update and draw functions.
MyGame.prototype.update = function() {
this.mResizeTest.setMousePosition(this.mCamera.mouseWCX(), this.mCamera.mouseWCY());
this.mResizeTest.update();
};
MyGame.prototype.draw = function () {
this.mResizeTest.draw(this.mCamera);
}